python学习lambda笔记
lamda 表达式的意义
# 需求:将列表中的元素按其平方进行排列
li = [3,5,-4,-1,0,-2,-6]
sorted(li, key=lambda x: x^2)
当然,也可以如下:
li1 = [3,5,-4,-1,0,-2,-6]
def get_square(x):
return (x^2)
sorted(list1,key=get_square)
充分显示了其 PEP
Explicit is better than implicit
的特点
“Talk is cheap. Show me the code.”
–Linus Torvalds
巨硬这回真的下功夫了——Edge 体验
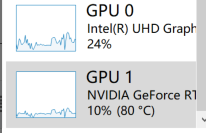
居然可以直接条用我的2070当web-gpu
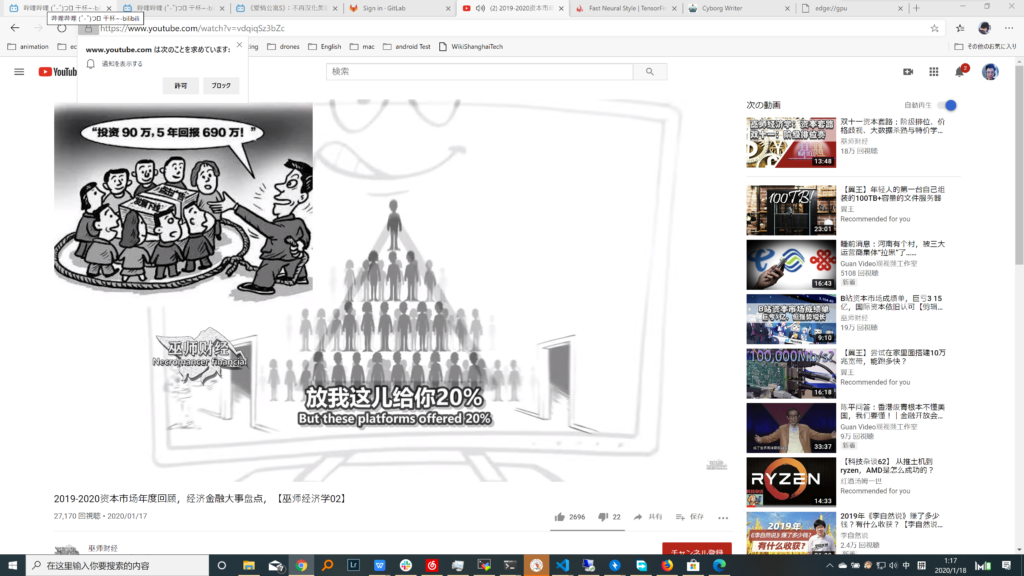
Graphics Feature Status
- Canvas: Hardware accelerated
- Flash: Hardware accelerated
- Flash Stage3D: Hardware accelerated
- Flash Stage3D Baseline profile: Hardware accelerated
- Compositing: Hardware accelerated
- Multiple Raster Threads: Enabled
- Out-of-process Rasterization: Disabled
- Hardware Protected Video Decode: Unavailable
- Rasterization: Hardware accelerated
- Skia Renderer: Disabled
- Video Decode: Hardware accelerated
- Viz Display Compositor: Enabled
- Viz Hit-test Surface Layer: Enabled
- WebGL: Hardware accelerated
- WebGL2: Hardware accelerated
Driver Bug Workarounds
- clear_uniforms_before_first_program_use
- decode_encode_srgb_for_generatemipmap
- disable_delayed_copy_nv12
- disable_direct_composition_video_overlays
- disable_discard_framebuffer
- disable_playready_hardware_drm
- disable_vp_scaling
- exit_on_context_lost
- force_cube_complete
- scalarize_vec_and_mat_constructor_args
- disabled_extension_GL_KHR_blend_equation_advanced
- disabled_extension_GL_KHR_blend_equation_advanced_coherent
Problems Detected
- Protected video decoding with swap chain is for Windows and Intel only
Disabled Features: protected_video_decode - Some drivers are unable to reset the D3D device in the GPU process sandbox
Applied Workarounds: exit_on_context_lost - Clear uniforms before first program use on all platforms: 124764, 349137
Applied Workarounds: clear_uniforms_before_first_program_use - Always rewrite vec/mat constructors to be consistent: 398694
Applied Workarounds: scalarize_vec_and_mat_constructor_args - ANGLE crash on glReadPixels from incomplete cube map texture: 518889
Applied Workarounds: force_cube_complete - Framebuffer discarding can hurt performance on non-tilers: 570897
Applied Workarounds: disable_discard_framebuffer - Disable KHR_blend_equation_advanced until cc shaders are updated: 661715
Applied Workarounds: disable(GL_KHR_blend_equation_advanced), disable(GL_KHR_blend_equation_advanced_coherent) - Decode and Encode before generateMipmap for srgb format textures on Windows: 634519
Applied Workarounds: decode_encode_srgb_for_generatemipmap - Delayed copy NV12 displays incorrect colors on NVIDIA drivers.: 728670
Applied Workarounds: disable_delayed_copy_nv12 - Hardware overlays result in black videos on non-Intel GPUs: 932879
Applied Workarounds: disable_direct_composition_video_overlays - Don't use video processor scaling on non-Intel GPUs.: 993233
Applied Workarounds: disable_vp_scaling - Don't use PlayReady hardware DRM on Turing-architecture NVIDIA GPUs.: 23413896
Applied Workarounds: disable_playready_hardware_drm
ANGLE Features
disable_program_caching_for_transform_feedback
(Frontend workarounds): Disabled
On some GPUs, program binaries don't contain transform feedback varyings
lose_context_on_out_of_memory
(Frontend workarounds): Enabled: true
Some users rely on a lost context notification if a GL_OUT_OF_MEMORY error occurs
scalarize_vec_and_mat_constructor_args
(Frontend workarounds)
398694
: Enabled: true
Always rewrite vec/mat constructors to be consistent
sync_framebuffer_bindings_on_tex_image
(Frontend workarounds): Disabled
On some drivers TexImage sometimes seems to interact with the Framebuffer
add_dummy_texture_no_render_target
(D3D workarounds)
anglebug:2152
: Disabled: isIntel && capsVersion < IntelDriverVersion(4815)
On some drivers when rendering with no render target, two bugs lead to incorrect behavior
allow_clear_for_robust_resource_init
(D3D workarounds)
941620
: Disabled: false
Some drivers corrupt texture data when clearing for robust resource initialization.
call_clear_twice
(D3D workarounds)
655534
: Disabled: isIntel && isSkylake && capsVersion < IntelDriverVersion(4771)
Using clear() may not take effect
depth_stencil_blit_extra_copy
(D3D workarounds)
anglebug:1452
: Disabled: (part1 <= 13u && part2 < 6881) && isNvidia && driverVersionValid
Bug in some drivers triggers a TDR when using CopySubresourceRegion from a staging texture to a depth/stencil
disable_b5g6r5_support
(D3D workarounds): Disabled: (isIntel && capsVersion < IntelDriverVersion(4539)) || isAMD
Textures with the format DXGI_FORMAT_B5G6R5_UNORM have incorrect data
emulate_isnan_float
(D3D workarounds)
650547
: Disabled: isIntel && isSkylake && capsVersion < IntelDriverVersion(4542)
Using isnan() on highp float will get wrong answer
emulate_tiny_stencil_textures
(D3D workarounds): Disabled: isAMD && !(deviceCaps.featureLevel < D3D_FEATURE_LEVEL_10_1)
1x1 and 2x2 mips of depth/stencil textures aren't sampled correctly
expand_integer_pow_expressions
(D3D workarounds): Enabled: true
The HLSL optimizer has a bug with optimizing 'pow' in certain integer-valued expressions
flush_after_ending_transform_feedback
(D3D workarounds): Enabled: isNvidia
Some drivers sometimes write out-of-order results to StreamOut buffers when transform feedback is used to repeatedly write to the same buffer positions
force_atomic_value_resolution
(D3D workarounds)
anglebug:3246
: Enabled: isNvidia
On some drivers the return value from RWByteAddressBuffer.InterlockedAdd does not resolve when used in the .yzw components of a RWByteAddressBuffer.Store operation
get_dimensions_ignores_base_level
(D3D workarounds): Enabled: isNvidia
Some drivers do not take into account the base level of the texture in the results of the HLSL GetDimensions builtin
mrt_perf_workaround
(D3D workarounds): Enabled: true
Some drivers have a bug where they ignore null render targets
pre_add_texel_fetch_offsets
(D3D workarounds): Disabled: isIntel
HLSL's function texture.Load returns 0 when the parameter Location is negative, even if the sum of Offset and Location is in range
rewrite_unary_minus_operator
(D3D workarounds): Disabled: isIntel && (isBroadwell || isHaswell) && capsVersion < IntelDriverVersion(4624)
Evaluating unary minus operator on integer may get wrong answer in vertex shaders
select_view_in_geometry_shader
(D3D workarounds): Disabled: !deviceCaps.supportsVpRtIndexWriteFromVertexShader
The viewport or render target slice will be selected in the geometry shader stage for the ANGLE_multiview extension
set_data_faster_than_image_upload
(D3D workarounds): Enabled: !(isIvyBridge || isBroadwell || isHaswell)
Set data faster than image upload
skip_vs_constant_register_zero
(D3D workarounds): Enabled: isNvidia
In specific cases the driver doesn't handle constant register zero correctly
use_instanced_point_sprite_emulation
(D3D workarounds): Disabled: isFeatureLevel9_3
Some D3D11 renderers do not support geometry shaders for pointsprite emulation
use_system_memory_for_constant_buffers
(D3D workarounds)
593024
: Disabled: isIntel
Copying from staging storage to constant buffer storage does not work
zero_max_lod
(D3D workarounds): Disabled: isFeatureLevel9_3
Missing an option to disable mipmaps on a mipmapped texture
Compositor Information
Tile Update Mode | One-copy |
Partial Raster | Enabled |
GpuMemoryBuffers Status
R_8 | Software only |
R_16 | Software only |
RG_88 | Software only |
BGR_565 | Software only |
RGBA_4444 | Software only |
RGBX_8888 | GPU_READ, SCANOUT |
RGBA_8888 | GPU_READ, SCANOUT |
BGRX_8888 | Software only |
BGRX_1010102 | Software only |
RGBX_1010102 | Software only |
BGRA_8888 | Software only |
RGBA_F16 | Software only |
YVU_420 | Software only |
YUV_420_BIPLANAR | Software only |
P010 | Software only |
Display(s) Information
Info | Display[2528732444] bounds=[0,0 1920x1080], workarea=[0,0 1920x1040], scale=2, external. |
Color space information | {primaries_d50_referred: [[0.6808, 0.3205], [0.2584, 0.7007], [0.1469, 0.0421]], transfer:IEC61966_2_1, matrix:RGB, range:FULL} |
SDR white level in nits | 80 |
Bits per color component | 8 |
Bits per pixel | 24 |
Refresh Rate in Hz | 59 |
Info | Display[2480450848] bounds=[-1920,0 1920x1080], workarea=[-1920,0 1920x1080], scale=1, external. |
Color space information | {primaries_d50_referred: [[0.6808, 0.3205], [0.2584, 0.7007], [0.1469, 0.0421]], transfer:IEC61966_2_1, matrix:RGB, range:FULL} |
SDR white level in nits | 80 |
Bits per color component | 8 |
Bits per pixel | 24 |
Refresh Rate in Hz | 60 |
Video Acceleration Information
Decode h264 baseline | 48x48 to 4096x2304 pixels |
Decode h264 baseline | 48x48 to 2304x4096 pixels |
Decode h264 main | 48x48 to 4096x2304 pixels |
Decode h264 main | 48x48 to 2304x4096 pixels |
Decode h264 high | 48x48 to 4096x2304 pixels |
Decode h264 high | 48x48 to 2304x4096 pixels |
Decode vp9 profile0 | 16x16 to 8192x8192 pixels |
Decode vp9 profile0 | 16x16 to 8192x8192 pixels |
Decode vp9 profile2 | 16x16 to 8192x8192 pixels |
Decode vp9 profile2 | 16x16 to 8192x8192 pixels |
Encode h264 baseline | 0x0 to 3840x2176 pixels, and/or 30.000 fps |
Encode h264 main | 0x0 to 3840x2176 pixels, and/or 30.000 fps |
Encode h264 high | 0x0 to 3840x2176 pixels, and/or 30.000 fps |
[Computer Architecture] Programming Counter
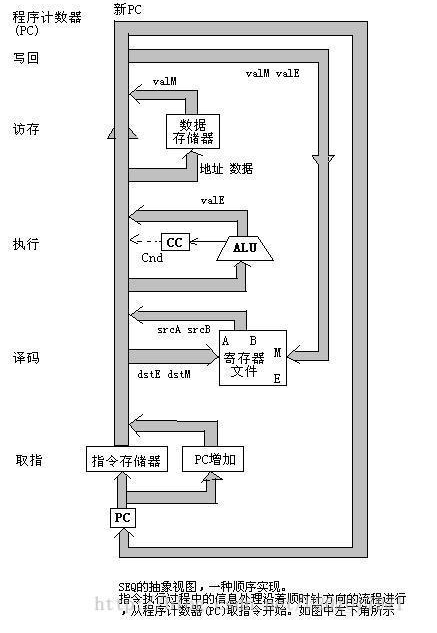
IBtest Failure resolution
之前是网断了,回机房插拔了一下就搞定了。
56Gbps延时是毫秒级别的!!
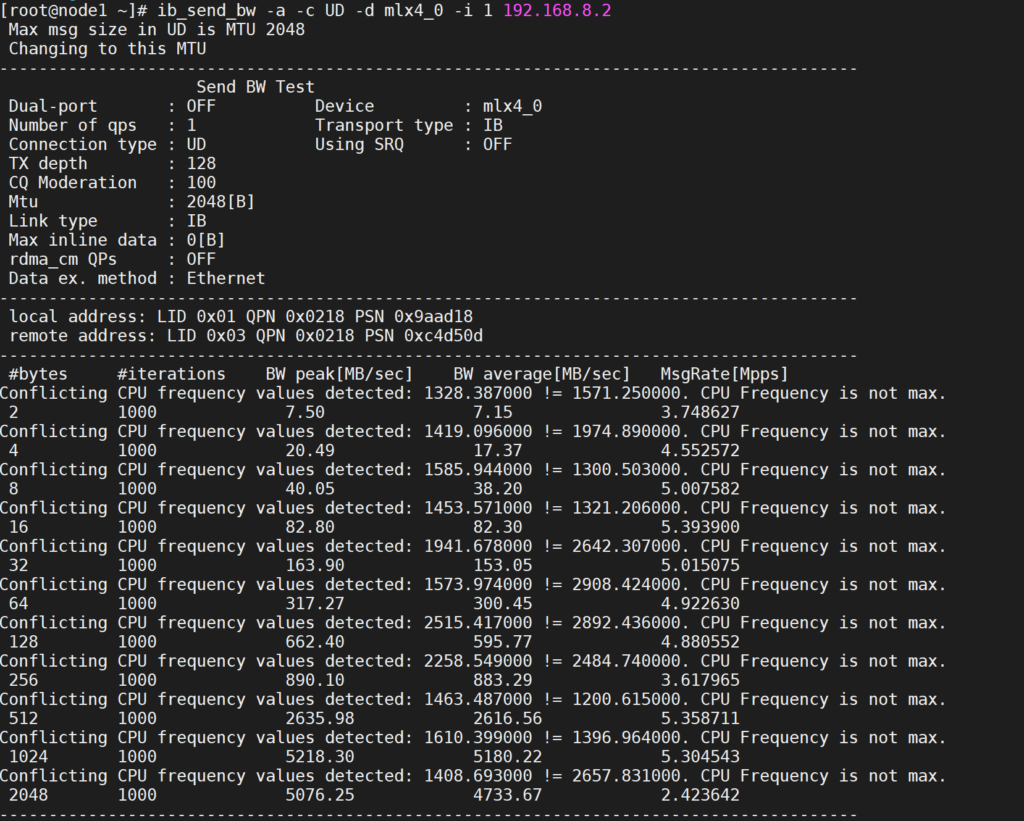
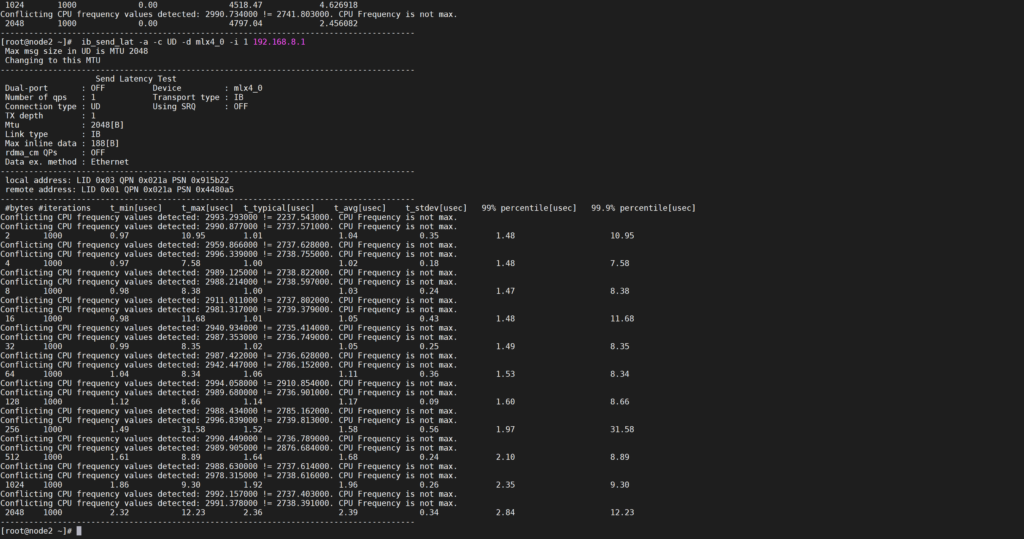
[学长的项目]测试"Detecting Adv from Artifacts" paper
看看开源世界的始末,还是觉得大家尤其是不一定要求源码的东西
QuEST test demo
machine

gcc no prefix
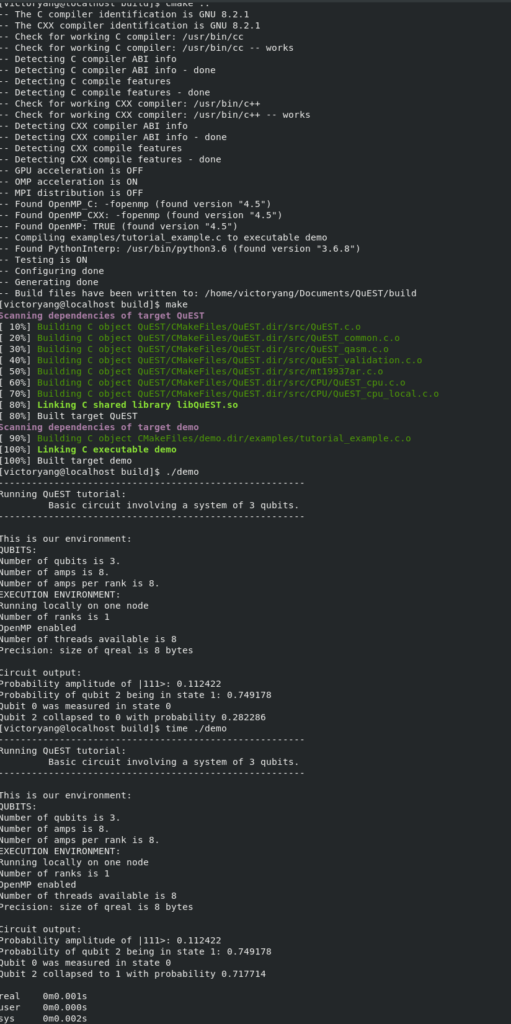

mpicc -ddistributed=1
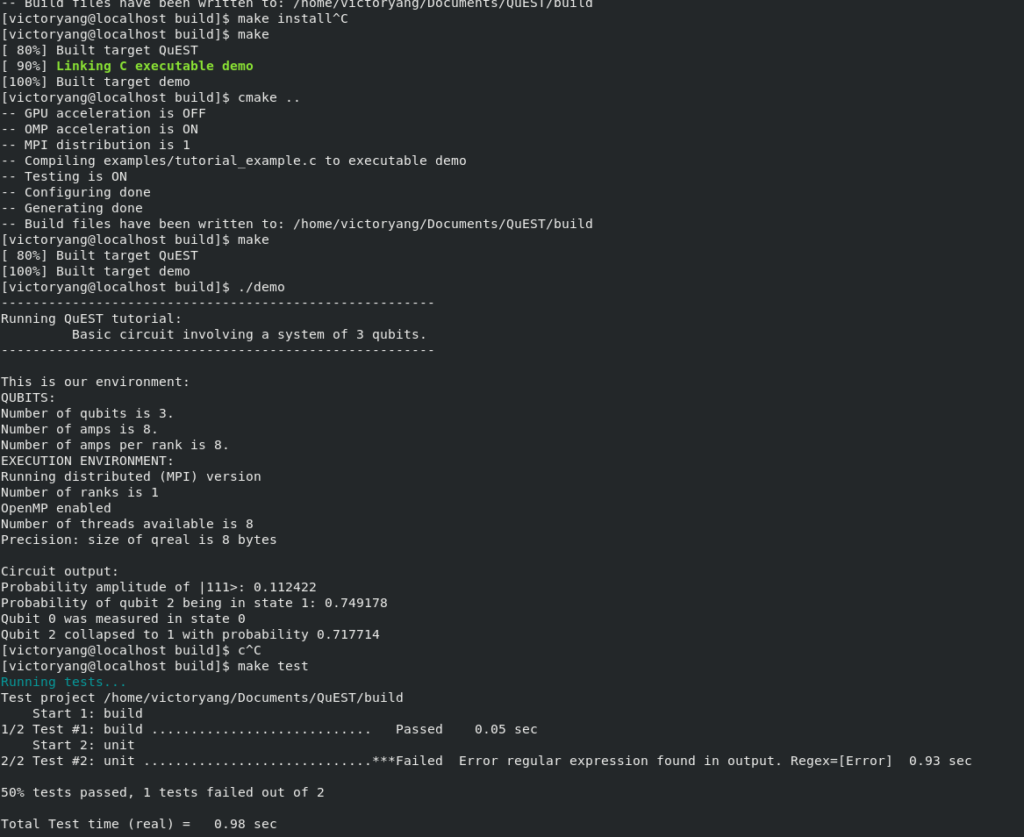
mpicc -ddistributed=2
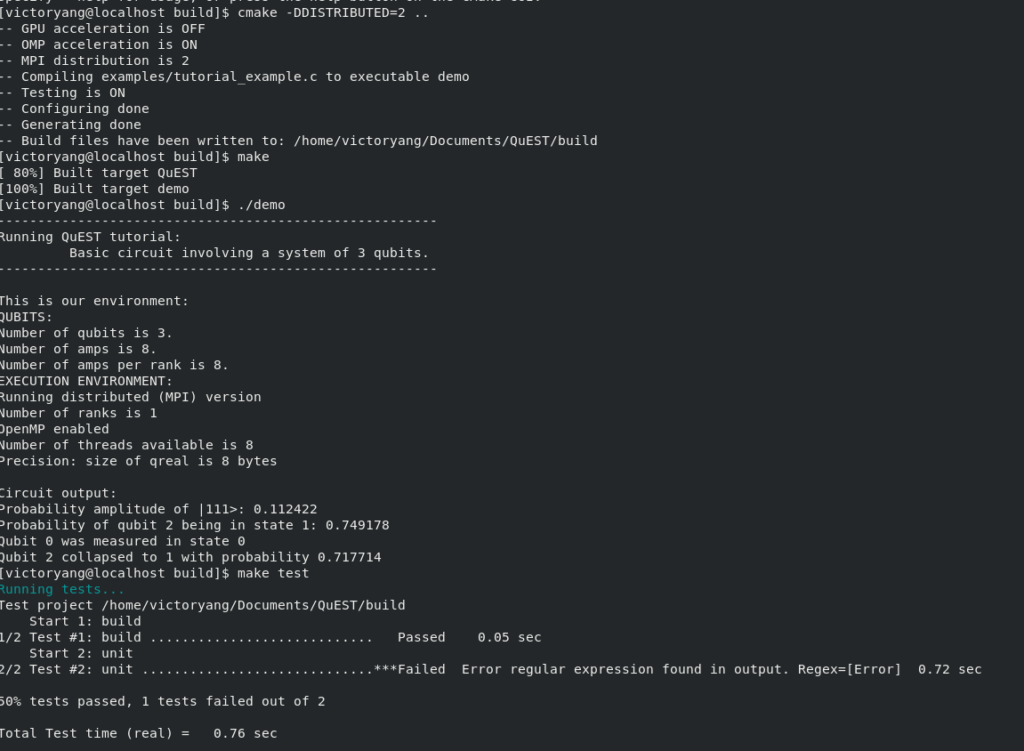
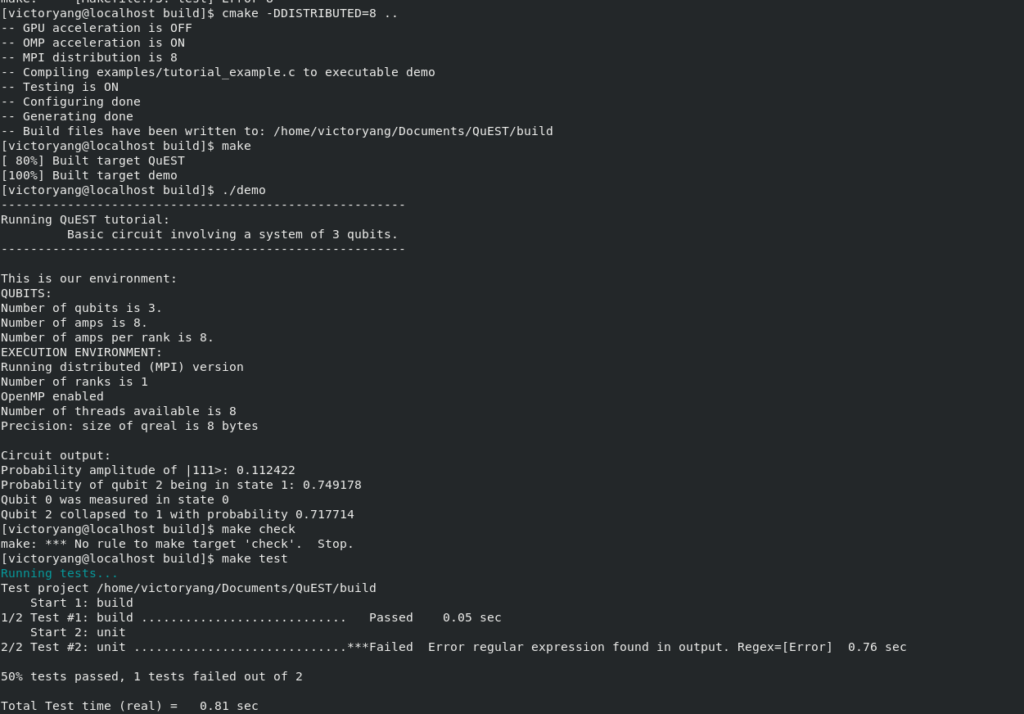
icc distribution=8
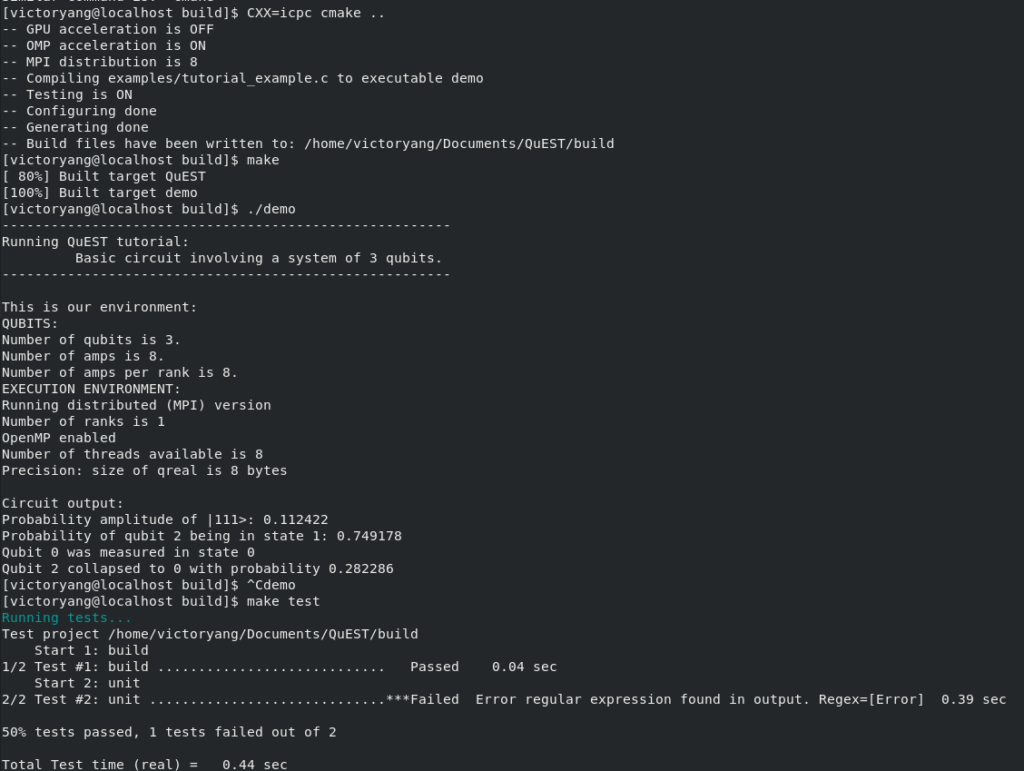
1080ti
gpu defaultly not compatible with parallel mpi
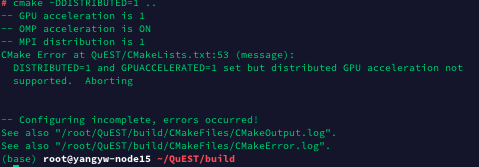