之前在CS100上了解到了工厂模式和Adaptor 模式
Factory Method
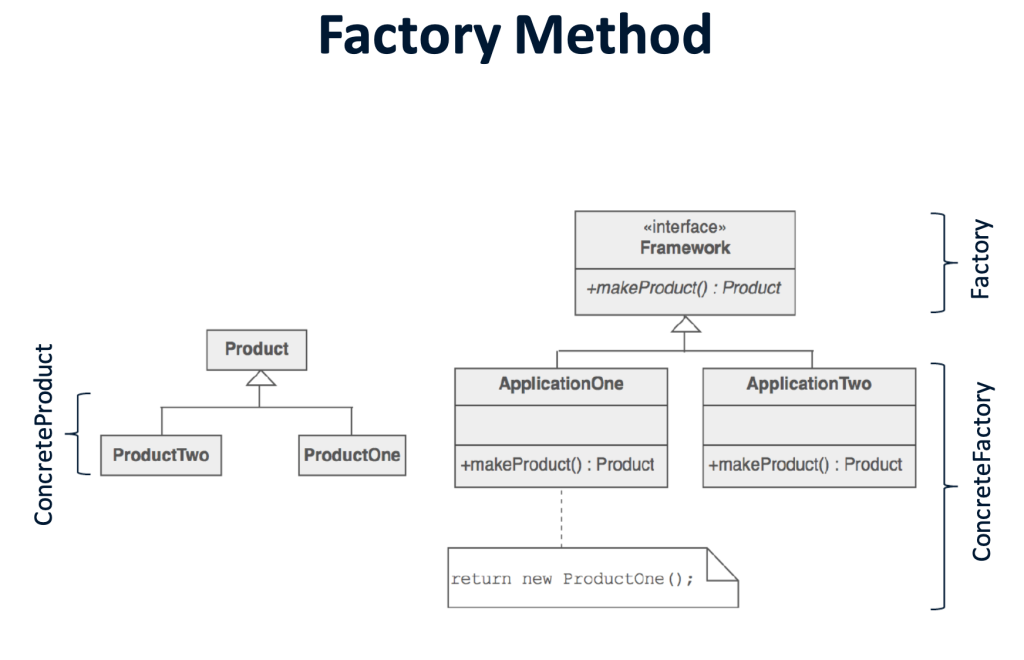
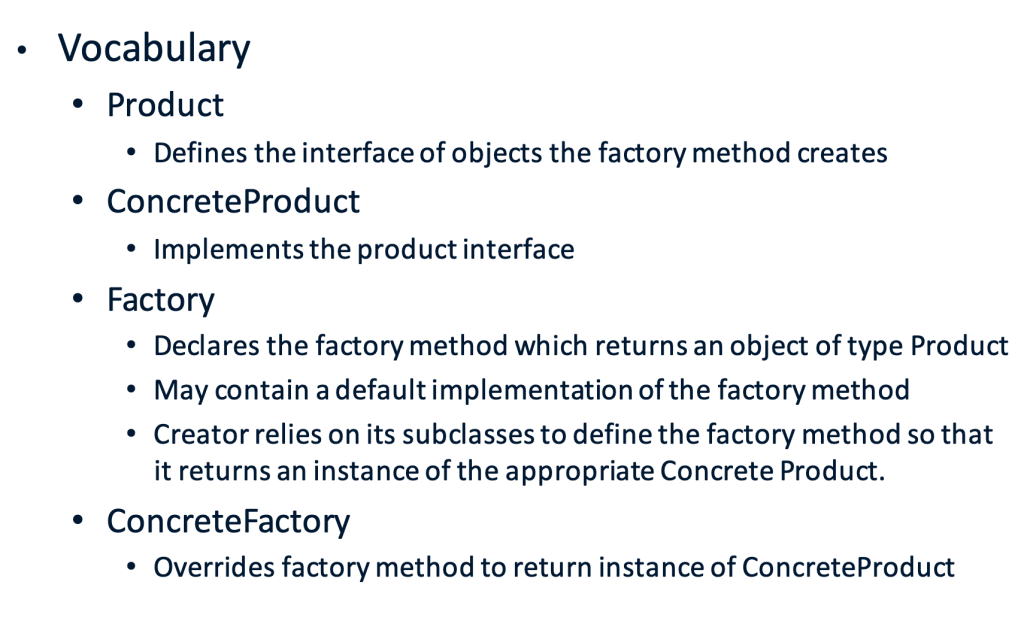
class Shape{
public:
static Shape * make_shape(int choice);
virtual void print() = 0; //纯虚函数
};
class Circle:public Shape{/*..*/ };
class Square:public Shape{/*..*/ };
class Rectangle:public Shape{/*..*/ };
Shape * Shape::make_shape(int choice) {
switch (choice) {
case 1:
return new Circle();
case 2:
return new Square();
case 3:
return new Rectangle();
}
}
后来写Compiler的时候觉得Factory Method可以用于传函数指针的代码生成器。
/*** Factory methods */
static string instConst(string (*inst)(const Reg &target, const Reg &op1, const Value &op2, string comment),
const Reg &target, const Reg &op1, const Constant &op2);
static string instConst(string (*inst)(const Reg &target, const Reg &op1, const Reg &op2, string comment),
const Reg &target, const Reg &op1, const Constant &op2);
static string instConst(string (*inst)(const Reg &target, const Reg &op1, int imm, string comment),
const Reg &target, const Reg &op1, const Constant &op2);
static string instConst(string (*inst)(const Reg &op1, const Value &op2, string comment), const Reg &op1,
const Constant &op2);
Adaptier Pattern
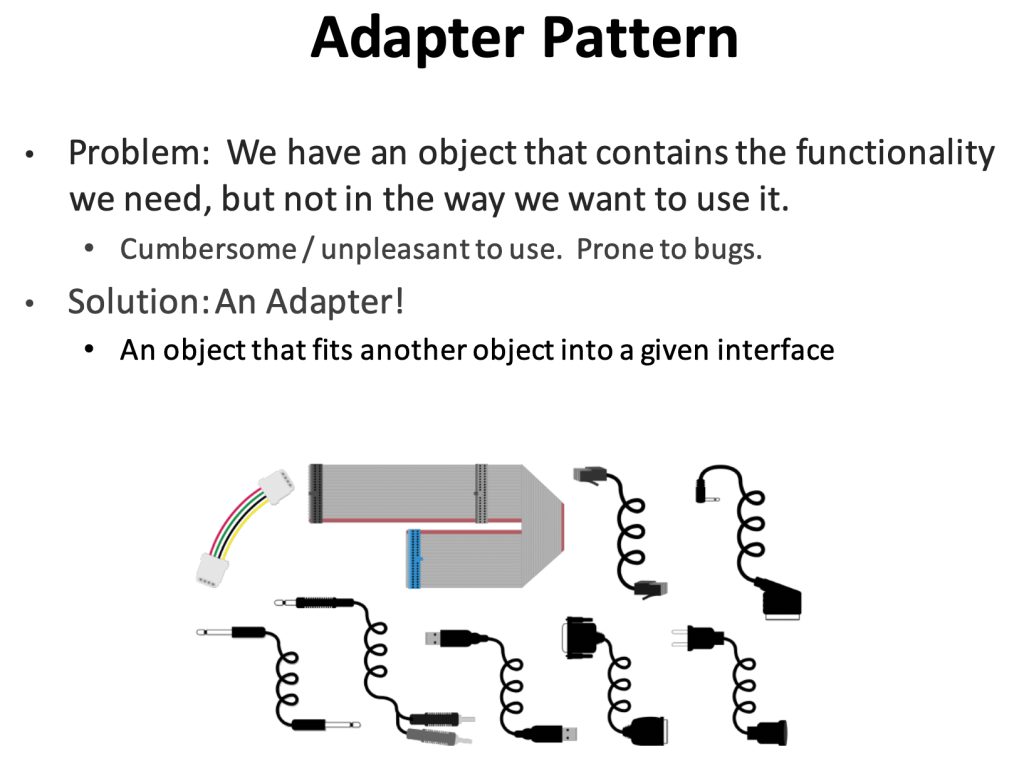
class LegacyRectangle{
public:
LegacyRectangle(float x1,float y1,float x2, float y2){
m_x1=x1; m_y1=y1; m_x2=x2; m_y2=y2;
}
float area(){
return (m_x2-m_x1)*(m_y2-m_y1);
}
private:
float m_x1;
float m_x2;
float m_y1;
float m_y2;
};
class Rectangle{
public:
virtual float areaSqInch(); //unit:square-inch
};
class RectangleAdapter:public Rectangle,private LegacyRectangle{
public:
RectangleAdapter(float x,float y,float w,float h): LegacyRectangle(x/39.27f,y/39.37f,(x+w)/39.37f,(y+h)/39.37f){}
virtual float areaSqInch(){
float areaSqM = area();
return areaSqM * 1550.0f;
}
};
用一个双向继承关系来完成Adaptor的功能。
这是在B站上设计模式那本书的总结
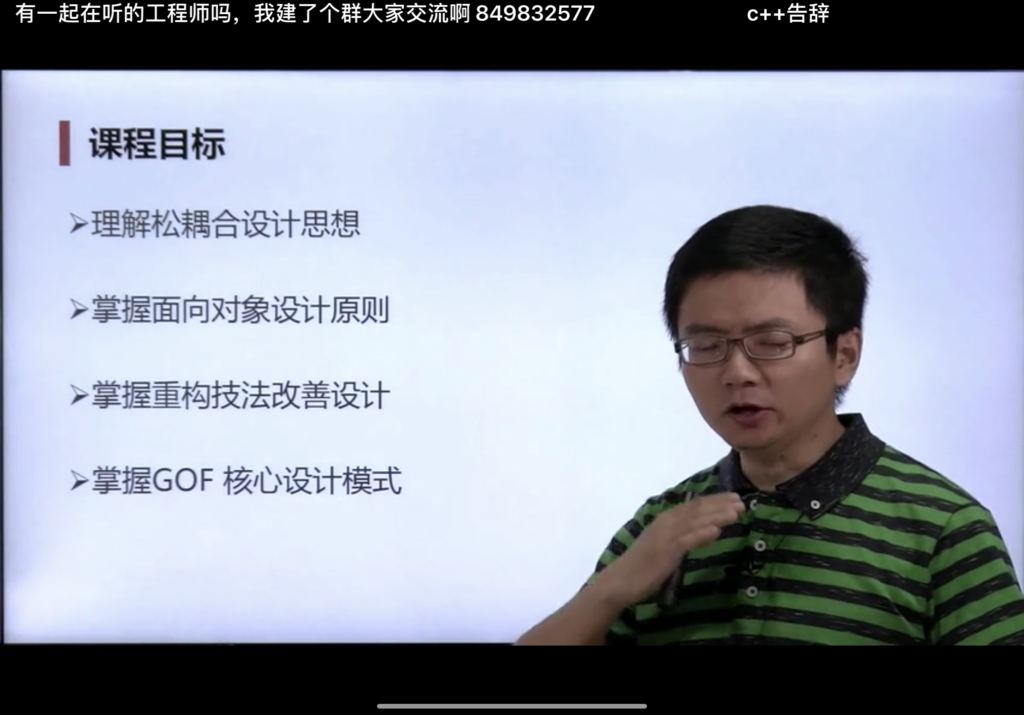
